股票技术指标源码是什么
```html
Stock Technical Indicators Source Code
Stock technical indicators are mathematical calculations based on historical price, volume, or open interest data. They are used by traders and analysts to make investment decisions and assess market trends. Here's an example source code in Python for calculating some common technical indicators:
import pandas as pd
import numpy as np
def moving_average(data, window):
return data.rolling(window=window).mean()
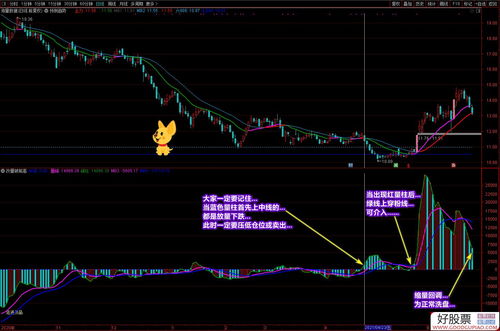
def exponential_moving_average(data, window):
return data.ewm(span=window, adjust=False).mean()
def relative_strength_index(data, window):
delta = data.diff(1)
gain = (delta.where(delta > 0, 0)).rolling(window=window).mean()
loss = (delta.where(delta < 0, 0)).rolling(window=window).mean()
rs = gain / loss
rsi = 100 (100 / (1 rs))
return rsi
def macd(data, short_window, long_window, signal_window):
short_ema = exponential_moving_average(data, short_window)
long_ema = exponential_moving_average(data, long_window)
macd_line = short_ema long_ema
signal_line = exponential_moving_average(macd_line, signal_window)
return macd_line, signal_line
Example usage:
data = pd.DataFrame({'Close': [100, 110, 120, 130, 140, 150, 160, 170, 180, 190]})
window = 3
short_window = 12
long_window = 26
signal_window = 9
data['SMA'] = moving_average(data['Close'], window)
data['EMA'] = exponential_moving_average(data['Close'], window)
data['RSI'] = relative_strength_index(data['Close'], window)
data['MACD'], data['Signal Line'] = macd(data['Close'], short_window, long_window, signal_window)
print(data)
This code snippet includes functions to calculate:
- Simple Moving Average (SMA)
- Exponential Moving Average (EMA)
- Relative Strength Index (RSI)
- Moving Average Convergence Divergence (MACD)
You can use this code as a starting point to develop more complex trading strategies or to analyze stock market data.